Message template to send media file
NOTE: DO NOT FORGET TO Add sample WHILE CREATING MEDIA TEMPLATE
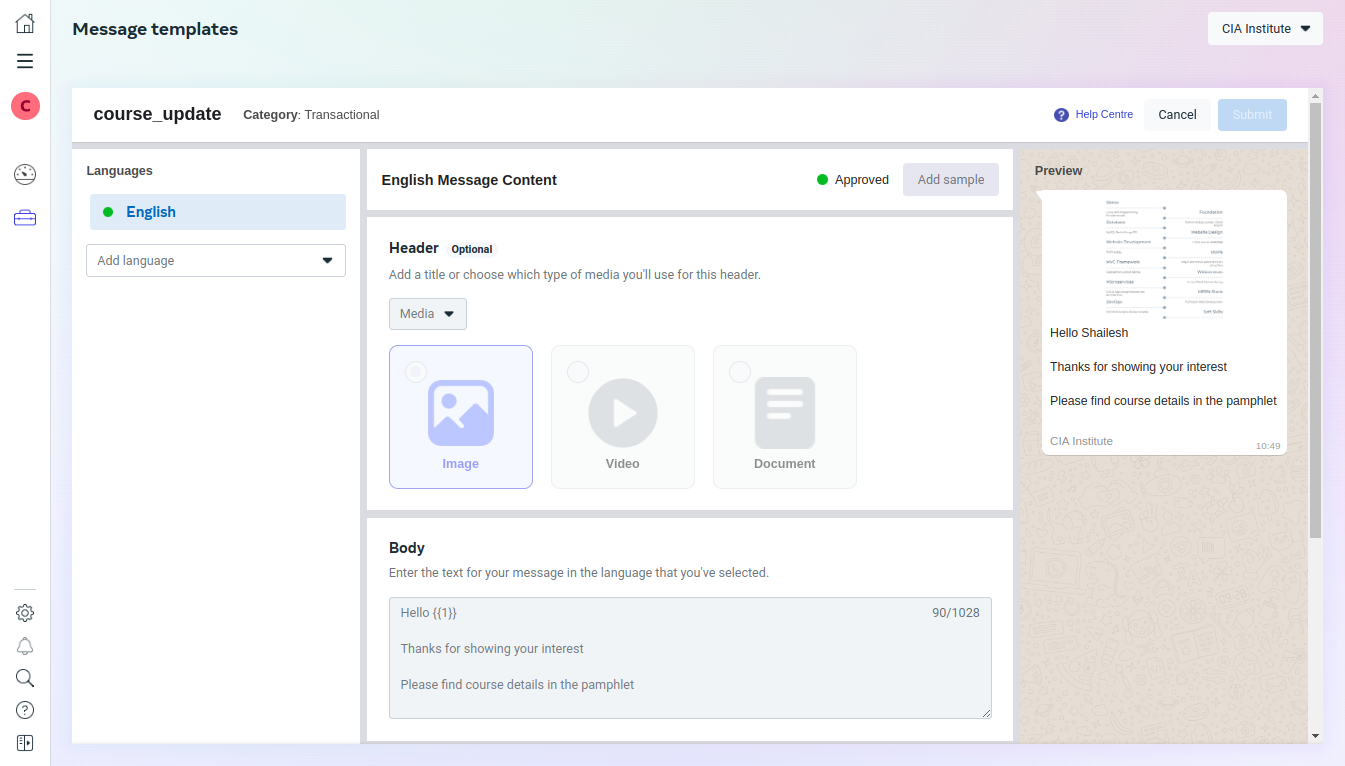
Upload media
<?php
$file="/tmp/test.jpg";
$mime = mime_content_type($file);
$info = pathinfo($file);
$name = $info['basename'];
$file = new CURLFile($file, $mime, $name);
$file->setMimeType($mime);
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://graph.facebook.com/{{Version}}/{{Phone-Number-ID}}/media",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_POSTFIELDS => array("messaging_product" => "whatsapp", "type"=>$mime, "file"=> $file),
CURLOPT_HTTPHEADER => array(
"Authorization: Bearer <YOUR TOKEN GOES HERE>"
),
));
$resultWhatsAppMedia = json_decode(curl_exec($curl), true);
$httpcode = curl_getinfo($curl, CURLINFO_HTTP_CODE);
$MEDIA_OBJECT_ID = $resultWhatsAppMedia['id']; //MEDIA OBJECT ID
echo $MEDIA_OBJECT_ID;
Payload to send media file
{
"messaging_product": "whatsapp",
"to": "91XXXXXXXXXX",
"type": "template",
"template": {
"name": "course_update",
"language": {
"code": "en_US"
},
"components": [{
"type": "header",
"parameters": [
{
"type": "image",
"image": {
"id": "XXXXXXXXXXXXXXXXXXX"
}
}
]
},{
"type": "body",
"parameters": [
{"type": "text", "text": "Shailesh"}
]
}]
}
}
Your message will look like this
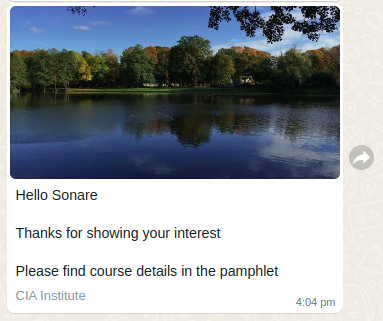
cURL call while sending message
curl -i -X POST https://graph.facebook.com/{{Version}}/{{Phone-Number-ID}}/messages -H 'Authorization: Bearer <YOUR TOKEN COMES HERE>' -H 'Content-Type: application/json' -d '{ "messaging_product": "whatsapp", "to": "91XXXXXXXXXX", "type": "template", "template": { "name": "hello_world", "language": { "code": "en_US" } } }'
Payload to send message with parameters
{
"messaging_product": "whatsapp",
"to": "91XXXXXXXXX",
"type": "template",
"template": {
"name": "full_payment",
"language": {
"code": "en_US"
},
"components": [{
"type": "body",
"parameters": [
{"type": "text", "text": "Shailesh"},
{"type": "text", "text": "5000"},
{"type": "text", "text": "https://urlgoeshere?id=12345"}
]
}]
}
cURL call with parameters
curl -i -X POST https://graph.facebook.com/{{Version}}/{{Phone-Number-ID}}/messages -H 'Authorization: Bearer <YOUR TOKEN COMES HERE>' -H 'Content-Type: application/json' -d '{ "messaging_product": "whatsapp", "to": "91XXXXXXXXXX", "type": "template", "template": { "name": "template_name", "language": { "code": "en_US" }, "components": [{ "type": "body", "parameters": [{"type": "text", "text": "<STRING VALUE>"},{"type": "text", "text": "₹ 7000"}]}] } }'
Manage Phone Numbers
https://business.facebook.com/wa/manage/phone-numbers/
Reference Links:
https://developers.facebook.com/docs/whatsapp/cloud-api/get-started/
https://developers.facebook.com/docs/whatsapp/api/messages/message-templates/media-message-templates/
https://developers.facebook.com/docs/whatsapp/message-templates/guidelines/