- Introduction – Difference between java and javascript and typescript and ecmascript
- Hello World in Chrome Console
- console.log() function
- alert() function
- console.trace() function
- Variables and datatypes
- Concatenation and Template Literals
- Difference between x, var x and let x
- document.getElementById
- document.getElementsByClassName
- document.querySelector
- value vs innerHTML
- Array – One dimensional and Multi dimensional
- Array Functions: forEach, map, reduce, filter, includes
- JSON object
- functions
- function with default arguments and …(spread operator / triple dots) argument
- setTimeout and setInterval function
- callback function
- lambda expression or arrow ()=>{} function in javascript
- events: onclick onkeypress onkeydown onkeyup onmouseover
- addEventListener(event, callBackFunction)
- function prototype
- class and objects using ECMA Script
- Object Destructuring
- Array of JSON
- AJAX using fetch API
Reference:
Assignments
- Basic (Solve any 5)
- Conditional: (Solve any 5)
if statement
switch statement - Loops (Solve any 5)
- Functions (Solve any 5)
- One Dimensional Array (Solve any 5)
- Two Dimensional Array (Solve any 5)
- OOPS (Solve any 5)
- WAP to create array of json having 10 records with key name age city and salary and print the records in HTML table
- WAP to find addition, subtraction, multiplication, division and modulus of two numbers using jQuery
- AJAX – WAP to fetch data from following REST API and show in HTML Table (User fetch and $.ajax)
1. https://jsonplaceholder.typicode.com/users/
2. http://dummy.restapiexample.com/api/v1/employees
3. https://reqres.in/api/users
4. https://api.randomuser.me/
Task: WAP to fetch data from google sheet
Ref Link: https://codeinsightacademy.com/blog/javascript/fetch-data-from-google-sheet/
Wireframe for assignment and task
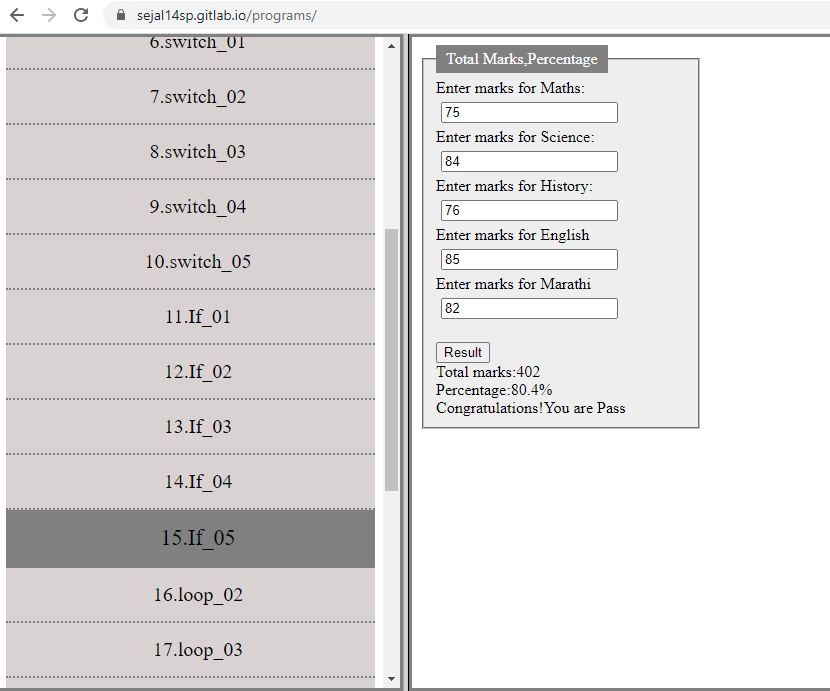
Ref Link: https://sejal14sp.gitlab.io/programs/