Step 1: Login to netlify.com from browser (preferably from default browser using github account)
Install netlify locally
npm install netlify-cli -g
Login to netlify from console
netlify init
Step 2: Create project locally with api file projectfolder/functions/api.js
const express = require('express');
const serverless = require('serverless-http');
const app = express();
const router = express.Router();
router.get('/', (req, res) => {
res.send('App is running..');
});
app.use('/.netlify/functions/api', router);
module.exports.handler = serverless(app);
//const port = 8080;
//app.listen(process.env.PORT || port, () => {
// console.log(`Listening on port ${port}`);
//});
Step 3: As we are deploying using lambda function create projectfolder/netlify.toml in project root directory
[build]
functions = "functions"
Step 4: Modify package.json file
{
"scripts": {
"build": "netlify deploy --prod"
},
"dependencies": {
"express": "^4.18.2",
"netlify-cli": "^12.7.2",
"netlify-lambda": "^2.0.15",
"serverless-http": "^3.2.0"
}
}
Step 5: Install required packages/modules
npm i express
Step 6: Test application locally
netlify functions:serve
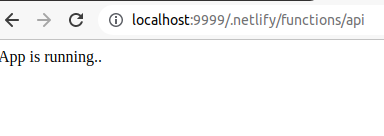
Step 7: Build the project and deploy on netlify
NOTE: If you are running it for 1st time then chose Create & configure a new site
npm run build
Access api from browser/postman
https://yourproject.netlify.app/.netlify/functions/api
You can check your functions api in netlify portal as well
https://app.netlify.com/sites/<your-project>/functions/api
CRUDL APP
api.js
const conn_str = "mongodb+srv://<username>:<password>@cluster0.<clusterid>.mongodb.net/<databasename>?retryWrites=true&w=majority";
const mongoose = require("mongoose");
mongoose.connect(conn_str)
.then(() => console.log("Connected successfully..."))
.catch( (error) => console.log(error) );
const express = require("express");
const serverless = require('serverless-http');
const app = express();
const router = express.Router();
var cors = require('cors')
app.use(express.json());
app.use(cors())
const empSchema = new mongoose.Schema( {
name: String,
contact_number: String,
address: String,
salary: Number,
employee_id: Number,
role: String
});
const emp = mongoose.models.emps || new mongoose.model("emps", empSchema);
router.get('/', (req, res) => {
res.send('App is running..');
});
router.get("/employees", async (req, res) => {
// var data = [{name: "hari", salary: 25000}, {name: "sameer", salary: 23000}]
let data = await emp.find();
res.send(data)
})
//fetch single document by id
//http://localhost:8989/employees/657d397eea713389134d1ffa
router.get("/employees/:id", async (req, res) => {
// console.log(req.params)
let data = await emp.find({_id: req.params['id']});
res.send(data[0])
})
//update document by id
router.put("/employees", async (req, res) => {
let u_data = await emp.updateOne({"_id": req.body.id}, {
"$set": {
"name" : req.body.name,
"salary" : req.body.salary,
}
});
res.send(u_data);
})
//http://localhost:8989/employees?id=657d397eea713389134d1ffe
router.delete("/employees", async (req, res) => {
let d_data = await emp.deleteOne({"_id": req.query['id']});
res.send(d_data);
})
router.post("/employees", async (req, res) => {
// doc = {
// "name":"harsha newly added",
// "contact_number":"9833910512",
// "address":"mumbai",
// "salary":20000,
// "employee_id":98829,
// "role":"operations"
// }
doc = req.body;
let u = await emp(doc);
let result = u.save();
res.send(doc);
})
app.use('/.netlify/functions/api', router);
module.exports.handler = serverless(app);