CalcController.java
package com.javatest.demo;
import org.springframework.web.bind.annotation.DeleteMapping;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.PutMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
class MyCalc {
private int num1;
private int num2;
public int getNum1() {
return num1;
}
public void setNum1(int num1) {
this.num1 = num1;
}
public int getNum2() {
return num2;
}
public void setNum2(int num2) {
this.num2 = num2;
}
@Override
public String toString() {
return "MyCalc [num1=" + num1 + ", num2=" + num2 + "]";
}
}
@RestController
public class CalcController {
@RequestMapping("test")
public String test() {
return "Testing....";
}
/** Read query params */
@RequestMapping("mycalc")
@GetMapping
public String getMet(@RequestParam("num1") int x, @RequestParam int y) {
return String.format("%s + %s = %s", x, y, x+y);
}
/** Read raw json data */
@PostMapping("mycalc")
public String postMet(@RequestBody MyCalc obj) {
int x = obj.getNum1();
int y = obj.getNum2();
return String.format("%s - %s = %s", x, y, x - y);
}
/** Read form data */
@PutMapping("mycalc")
public String putMet(
@RequestParam("num1") int x,
@RequestParam("num2") int y) {
return String.format("%s * %s = %s", x, y, x * y);
}
/** Read from raw json */
@DeleteMapping("mycalc")
public String deleteMet(@RequestBody MyCalc obj) {
int x = obj.getNum1();
int y = obj.getNum2();
return String.format("%s / %s = %s", x, y, x / y);
}
}
src/main/resources/application.properties
spring.datasource.url=jdbc:mysql://localhost:3306/<databasename>
spring.datasource.username=<username>
spring.datasource.password=<password>
spring.datasource.driverClassName=com.mysql.cj.jdbc.Driver
User.java (POJO/DAO/JPA)
package com.javatest.demo;
import jakarta.persistence.Entity;
import jakarta.persistence.GeneratedValue;
import jakarta.persistence.GenerationType;
import jakarta.persistence.Id;
import jakarta.persistence.Table;
@Entity
@Table(name="users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String age;
private String city;
private int quota;
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAge() {
return age;
}
public void setAge(String age) {
this.age = age;
}
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
public int getQuota() {
return quota;
}
public void setQuota(int quota) {
this.quota = quota;
}
// getters and setters
}
UserController.java
package com.javatest.demo;
import java.util.List;
import org.springframework.web.bind.annotation.DeleteMapping;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.PutMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.data.jpa.repository.JpaRepository;
interface UserRepository extends JpaRepository<User, Long> {
// Custom queries can be defined here
}
@RestController
@RequestMapping("users")
public class UserController {
private final UserRepository userRepository;
public UserController(UserRepository userRepository) {
this.userRepository = userRepository;
}
@RequestMapping("")
public String index() {
return "I am from index";
}
@RequestMapping("listing")
public List<User> listing() {
// List<User> users = new ArrayList<>();
return userRepository.findAll();
}
@DeleteMapping("delete/{id}")
public String deleteUser(@PathVariable Long id) {
// Check if the user with the specified ID exists
if (userRepository.existsById(id)) {
userRepository.deleteById(id);
return String.format("User %s is deleted successfully", id);
} else {
// Handle the case when the user does not exist (e.g., return an error response)
// You can throw an exception or return an appropriate response based on your application's requirements.
}
return "";
}
@PostMapping("create")
public User createUser(@RequestBody User user) {
return userRepository.save(user);
}
@GetMapping("get-single/{id}")
public User getUserById(@PathVariable Long id) {
// Use the UserRepository to fetch the user by ID
return userRepository.findById(id).orElse(null);
}
@PutMapping("/update/{id}")
public User updateUser(@PathVariable Long id, @RequestBody User updatedUser) {
// Check if the user with the specified ID exists
userRepository.findById(id).orElse(null);
// Set the ID of the updated user to the specified ID
updatedUser.setId(id);
// Save the updated user to the database
return userRepository.save(updatedUser);
}
}
DemoApplication.java (Run this file to serve spring boot application)
package com.javatest.demo;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
File Structure
.
├── HELP.md
├── mvnw
├── mvnw.cmd
├── pom.xml
├── src
│ ├── main
│ │ ├── java
│ │ │ └── com
│ │ │ └── javatest
│ │ │ └── demo
│ │ │ ├── CalcController.java
│ │ │ ├── DemoApplication.java
│ │ │ ├── User.java
│ │ │ └── UserController.java
│ │ └── resources
│ │ ├── application.properties
│ │ ├── static
│ │ └── templates
│ └── test
│ └── java
│ └── com
│ └── javatest
│ └── demo
│ └── DemoApplicationTests.java
└── target
├── classes
│ ├── application.properties
│ └── com
│ └── javatest
│ └── demo
│ ├── CalcController.class
│ ├── DemoApplication.class
│ ├── MyCalc.class
│ ├── User.class
│ ├── UserController.class
│ └── UserRepository.class
└── test-classes
└── com
└── javatest
└── demo
└── DemoApplicationTests.class
23 directories, 18 files
POC
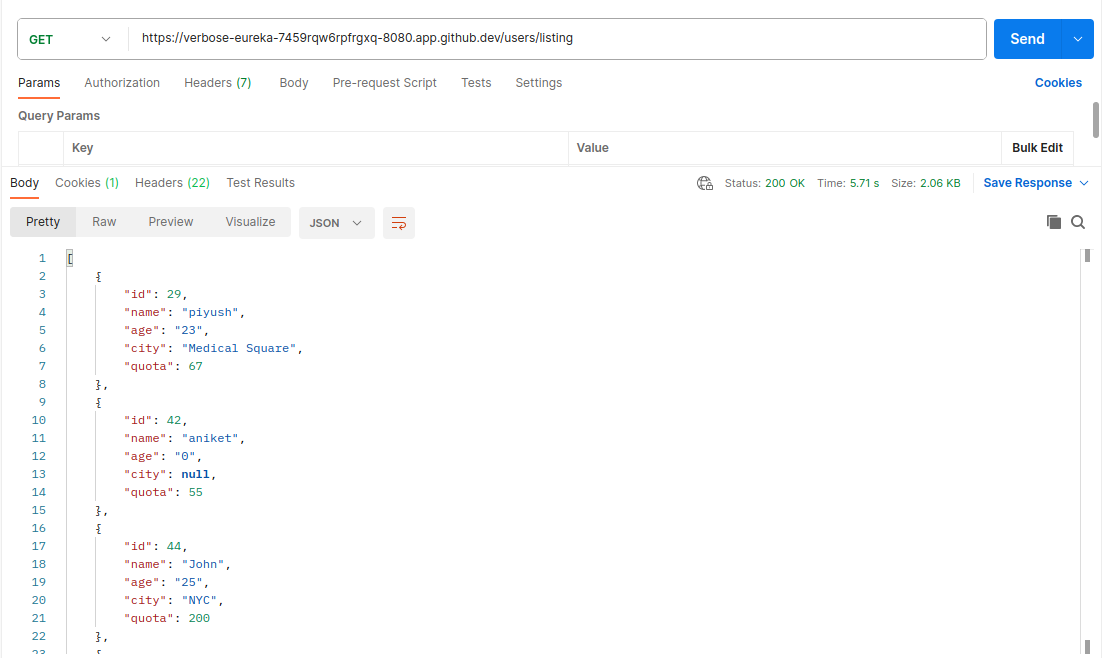
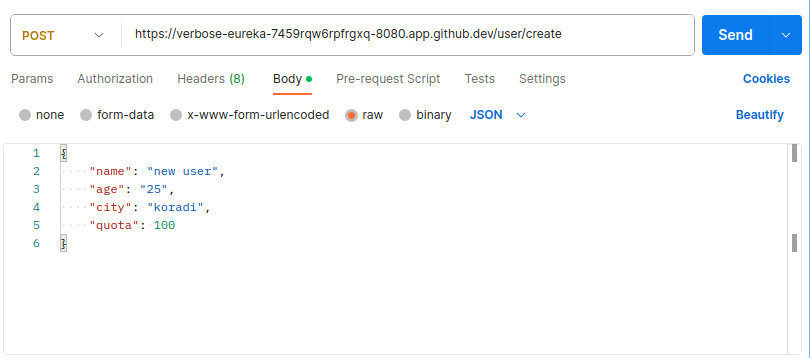
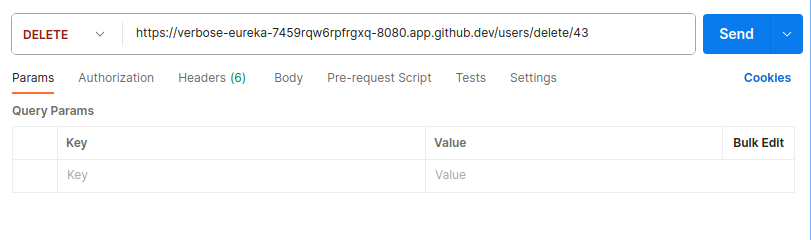
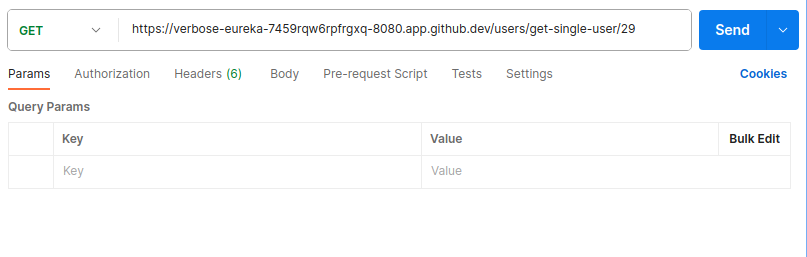
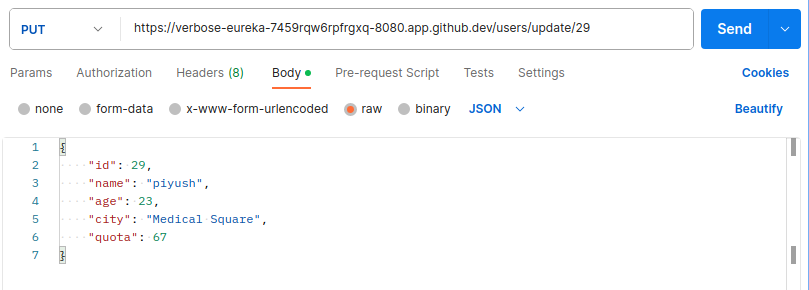
VSCode Extesnsions
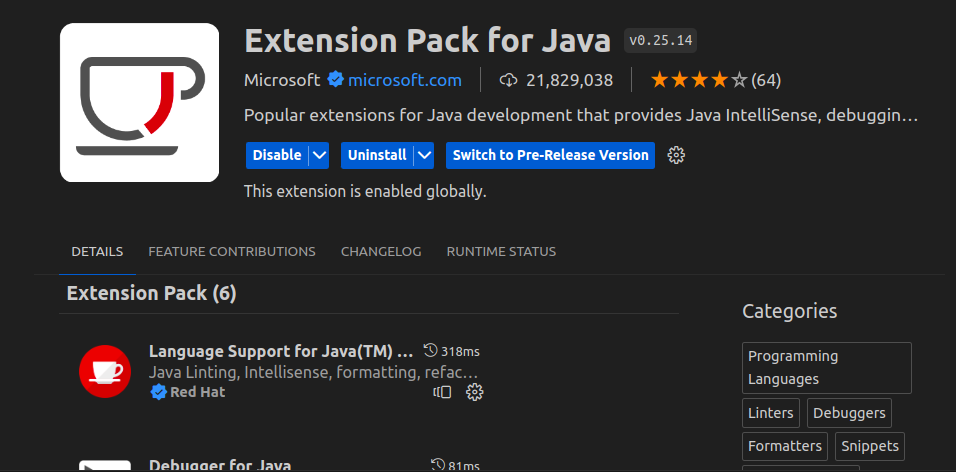
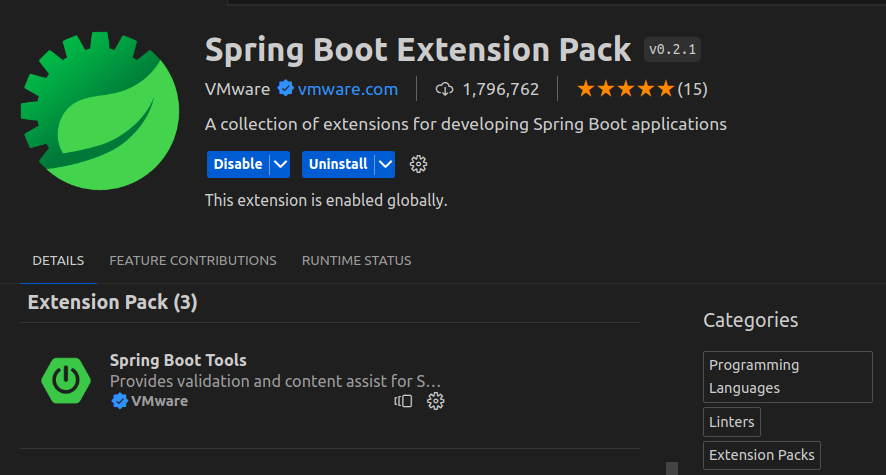