Certainly! Here are ten examples for each of the topics you mentioned:
BASICS
- Basics:
Example 1: Printing a message
print("Hello, World!")
Example 2: Arithmetic operations
a = 10 b = 5 print("Addition:", a + b) print("Subtraction:", a - b) print("Multiplication:", a * b) print("Division:", a / b) print("Modulo:", a % b)
Example 3: String concatenation
name = "Alice" age = 25 print("My name is " + name + " and I am " + str(age) + " years old.")
Example 4: Using the input function
name = input("Enter your name: ") print("Hello, " + name + "!")
Example 5: Conditional statements
num = int(input("Enter a number: ")) if num > 0: print("The number is positive.") elif num < 0: print("The number is negative.") else: print("The number is zero.")
Example 6: Working with loops (for loop)
for i in range(5): print(i)
Example 7: Working with loops (while loop)
count = 0 while count < 5: print(count) count += 1
Example 8: Using the len() function
text = "Hello, World!" print("Length:", len(text))
Example 9: Using the str() function
num = 42 text = "The answer is: " + str(num) print(text)
Example 10: Importing and using modules
import math radius = 5 area = math.pi * radius ** 2 print("Area of the circle:", area)
CONDITIONAL STATEMENTS IF ELSE
- If-Else Statements:
Example 1: Checking if a number is even or odd
num = int(input("Enter a number: ")) if num % 2 == 0: print("The number is even.") else: print("The number is odd.")
Example 2: Checking if a year is a leap year
year = int(input("Enter a year: ")) if year % 400 == 0 or (year % 4 == 0 and year % 100 != 0): print("The year is a leap year.") else: print("The year is not a leap year.")
Example 3: Determining the maximum of three numbers
num1 = float(input("Enter the first number: ")) num2 = float(input("Enter the second number: ")) num3 = float(input("Enter the third number: ")) max_num = max(num1, num2, num3) print("The maximum number is:", max_num)
Example 4: Checking if a student passed or failed
score = float(input("Enter the student's score: ")) if score >= 60: print("The student passed.") else: print("The student failed.")
Example 5
: Categorizing a number into different ranges
num = float(input("Enter a number: ")) if num < 0: print("The number is negative.") elif num >= 0 and num <= 10: print("The number is between 0 and 10.") elif num > 10 and num <= 20: print("The number is between 10 and 20.") else: print("The number is greater than 20.")
Example 6: Checking if a person is eligible to vote
age = int(input("Enter your age: ")) if age >= 18: print("You are eligible to vote.") else: print("You are not eligible to vote yet.")
Example 7: Checking if a number is positive, negative, or zero (alternative approach)
num = float(input("Enter a number: ")) if num > 0: print("The number is positive.") elif num < 0: print("The number is negative.") else: print("The number is zero.")
Example 8: Checking if a character is a vowel or consonant
char = input("Enter a character: ").lower() if char in ['a', 'e', 'i', 'o', 'u']: print("The character is a vowel.") else: print("The character is a consonant.")
Example 9: Checking if a number is a multiple of another number
num1 = int(input("Enter the first number: ")) num2 = int(input("Enter the second number: ")) if num1 % num2 == 0: print(num1, "is a multiple of", num2) else: print(num1, "is not a multiple of", num2)
Example 10: Checking if a year is a leap year (alternative approach)
year = int(input("Enter a year: ")) if year % 400 == 0 or (year % 4 == 0 and year % 100 != 0): print("The year is a leap year.") else: print("The year is not a leap year.")
For loop with range
- Printing numbers from 0 to 9:
for i in range(10): print(i)
- Printing even numbers from 2 to 10:
for i in range(2, 11, 2): print(i)
- Calculating the sum of numbers from 1 to 100:
total = 0 for i in range(1, 101): total += i print("Sum:", total)
- Printing numbers in reverse order from 9 to 0:
for i in range(9, -1, -1): print(i)
- Multiplying each number in the range by 2 and printing the result:
for i in range(10): result = i * 2 print(result)
- Printing the square of each number in the range from 1 to 5:
for i in range(1, 6): square = i ** 2 print(square)
- Printing numbers in increments of 5 from 0 to 50:
for i in range(0, 51, 5): print(i)
- Checking if a number is divisible by 3 in the range from 1 to 20:
for i in range(1, 21): if i % 3 == 0: print(i, "is divisible by 3")
- Printing the ASCII value of each character in a string:
text = "Hello" for char in text: ascii_value = ord(char) print(char, ":", ascii_value)
- Repeating a specific action a certain number of times using range:
for _ in range(5): print("Hello, world!")
LIST
- List:
Example 1: Accessing list elements
fruits = ["apple", "banana", "orange", "grape", "mango"] print(fruits[0]) # "apple" print(fruits[2]) # "orange"
Example 2: Modifying list elements
numbers = [1, 2, 3, 4, 5] numbers[2] = 10 print(numbers) # [1, 2, 10, 4, 5]
Example 3: Appending elements to a list
numbers = [1, 2, 3] numbers.append(4) print(numbers) # [1, 2, 3, 4]
Example 4: Removing elements from a list
fruits = ["apple", "banana", "orange", "grape"] fruits.remove("banana") print(fruits) # ["apple", "orange", "grape"]
Example 5: Slicing a list
numbers = [1, 2, 3, 4, 5] print(numbers[1:4]) # [2, 3, 4]
Example 6: Checking if an element exists in a list
fruits = ["apple", "banana", "orange", "grape"] if "banana" in fruits: print("Banana is in the list.")
Example 7: Counting occurrences of an element in a list
numbers = [1, 2, 3, 1, 2, 1, 4, 1] count = numbers.count(1) print("Number of occurrences:", count)
Example 8: Sorting a list
numbers = [5, 3, 1, 4, 2] numbers.sort() print(numbers) # [1, 2, 3, 4, 5]
Example 9: Reversing a list
fruits = ["apple", "banana", "orange", "grape"] fruits.reverse() print(fruits) # ["grape", "orange", "banana", "apple"]
Example 10: Combining two lists
list1 = [1, 2, 3] list2 = [4, 5, 6] combined_list = list1 + list2 print(combined_list) # [1, 2, 3, 4, 5, 6]
DICTIONARY
- Example 1: Accessing dictionary values
student = {"name": "Alice", "age": 20, "grade": "A"} print(student["name"]) # "Alice" print(student["age"]) # 20
Example 2: Adding new key-value pairs to a dictionary
student = {"name": "Alice", "age": 20} student["grade"] = "A" print(student) # {"name": "Alice", "age": 20, "grade": "A"}
Example 3: Modifying dictionary values
student = {"name": "Alice", "age": 20, "grade": "A"} student["age"] = 21 print(student) # {"name": "Alice", "age": 21, "grade": "A"}
Example 4: Checking if a key exists in a dictionary
student = {"name": "Alice", "age": 20, "grade": "A"} if "age" in student: print("Age:", student["age"]) # Age: 20
Example 5: Removing a key-value pair from a dictionary
student = {"name": "Alice", "age": 20, "grade": "A"} del student["grade"] print(student) # {"name": "Alice", "age": 20}
Example 6: Getting all keys from a dictionary
student = {"name": "Alice", "age": 20, "grade": "A"} keys = student.keys() print(keys) # ["name", "age", "grade"]
Example 7: Getting all values from a dictionary
student = {"name": "Alice", "age": 20, "grade": "A"} values = student.values() print(values) # ["Alice", 20, "A"]
Example 8: Checking the length of a dictionary
student = {"name": "Alice", "age": 20, "grade": "A"} length = len(student) print("Length:", length) # Length: 3
Example 9: Clearing a dictionary
student = {"name": "Alice", "age": 20, "grade": "A"} student.clear() print(student) # {}
Example 10: Copying a dictionary
student = {"name": "Alice", "age": 20, "grade": "A"} student_copy = student.copy() print(student_copy) # {"name": "Alice", "age": 20, "grade": "A"}
LIST OF DICTIONARIES
employees = [ {"name": "John", "age": 32, "department": "HR", "salary": 50000}, {"name": "Emily", "age": 28, "department": "IT", "salary": 60000}, {"name": "Michael", "age": 35, "department": "Finance", "salary": 70000}, {"name": "Sophia", "age": 29, "department": "Sales", "salary": 55000}, {"name": "Daniel", "age": 31, "department": "IT", "salary": 62000}, {"name": "Olivia", "age": 27, "department": "HR", "salary": 48000}, {"name": "William", "age": 33, "department": "Finance", "salary": 75000}, {"name": "Ava", "age": 30, "department": "Sales", "salary": 58000}, {"name": "James", "age": 34, "department": "IT", "salary": 65000}, {"name": "Emma", "age": 26, "department": "HR", "salary": 52000} ]
Now, let’s provide 10 examples using the same list of employees:
- Accessing values in the list:
print(employees[0]["name"]) # Output: "John" print(employees[3]["age"]) # Output: 29
- Modifying a value in the list:
employees[2]["salary"] = 72000 print(employees[2]) # Output: {'name': 'Michael', 'age': 35, 'department': 'Finance', 'salary': 72000}
- Adding a new key-value pair to a dictionary:
employees[1]["position"] = "Senior Software Engineer" print(employees[1]) # Output: {'name': 'Emily', 'age': 28, 'department': 'IT', 'salary': 60000, 'position': 'Senior Software Engineer'}
- Removing a key-value pair from a dictionary:
del employees[4]["age"] print(employees[4]) # Output: {'name': 'Daniel', 'department': 'IT', 'salary': 62000}
- Counting the number of dictionaries in the list:
count = len(employees) print("Number of employees:", count) # Output: Number of employees: 10
- Calculating the average age of all employees:
total_salary = 0 num_employees = len(employees) for employee in employees: total_salary += employee["salary"] average_salary = total_salary / num_employees print("Average salary:", average_salary)
- Finding the employee with the highest salary:
max_salary = 0 employee_with_max_salary = None for employee in employees: if employee["salary"] > max_salary: max_salary = employee["salary"] employee_with_max_salary = employee print("Employee with the highest salary:") print("Name:", employee_with_max_salary["name"]) print("Salary:", employee_with_max_salary["salary"])
- Finding all employees having highest salary:
max_salary = 0 employees_with_max_salary = [] for employee in employees: if employee["salary"] > max_salary: max_salary = employee["salary"] employees_with_max_salary = [employee] elif employee["salary"] == max_salary: employees_with_max_salary.append(employee) print("Employees with the highest salary:") for employee in employees_with_max_salary: print("Name:", employee["name"]) print("Salary:", employee["salary"]) print()
- Calculating total, average, highest and lowest salaries all employees:
total_salary = 0 highest_salary = float("-inf") lowest_salary = float("inf") for employee in employees: salary = employee["salary"] total_salary += salary if salary > highest_salary: highest_salary = salary if salary < lowest_salary: lowest_salary = salary average_salary = total_salary / len(employees) print("Total Salary:", total_salary) print("Highest Salary:", highest_salary) print("Lowest Salary:", lowest_salary) print("Average Salary:", average_salary)
- Print all employees using PrettyTable:
# Print all employees all_employees_table = PrettyTable(["Name", "Department", "Salary"]) for employee in employees: all_employees_table.add_row([employee["name"], employee["department"], employee["salary"]]) all_employees_table.title = "All Employees" print(all_employees_table)
Mini Project
Print department wise highest salaried employees
Expected output
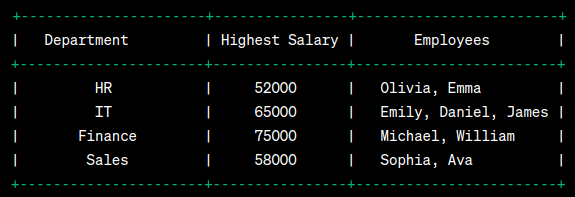