To install phpunit framework make sure you have installed composer
If not follow instructions from this link http://codeinsightacademy.com/blog/php/composer/
Installation
Install php-xml
As we are going to use phpunit.xml we need to install php-xml
sudo apt install php-xml
#To install specific version
sudo apt install php7.4-xml
Install mbstring
sudo apt-get install php-mbstring
Create composer.json file in project root directory
{
"autoload" : {}
}
Run one of the composer command
composer dump-autoload -o
OR
composer update
Install sluggable
composer require cviebrock/eloquent-sluggable
Install intl
This is required when you are using Code Igniter framework
apt-get install php-intl
#OR specific version
apt-get install php7.4-intl
Install php unit framework
composer require phpunit/phpunit ^9
Create function
global_functions.php
<?php
function add($x = 0, $y = 0) {
return $x + $y;
}
index.php
<?php
require_once("vendor/autoload.php");
echo add(5, 6);
composer.json
{
"autoload": {
"files": ["global_functions.php"]
},
"require": {
"cviebrock/eloquent-sluggable": "^8.0",
"phpunit/phpunit": "^9"
}
}
Run index.php file
php index.php
Write Testcase
phpunit.xml
<?xml version="1.0" encoding="UTF-8"?>
<phpunit bootstrap = "vendor/autoload.php"
colors = "true">
<testsuites>
<testsuite name="Sample test suite">
<directory>tests</directory>
</testsuite>
</testsuites>
</phpunit>
tests/GlobalFunctionsTest.php
NOTE: MAKE SURE TO GIVE test PREFIX TO YOUR TEST FUNCTION
<?php
class GlobalFunctionsTest extends \PHPUnit\Framework\TestCase {
public function testAdd() {
$result = add(5, 6);
$this->assertEquals(11, $result);
}
}
Get all assertions functions from here
https://phpunit.readthedocs.io/en/9.5/assertions.html
Run TestCase from root directory
./vendor/bin/phpunit
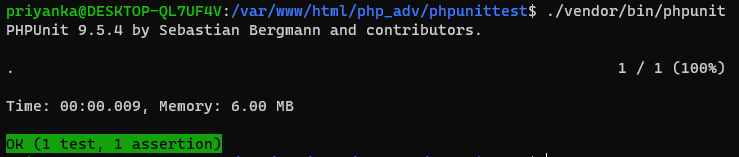
./vendor/bin/phpunit --testdox
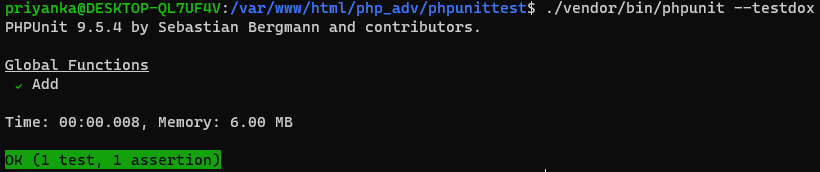
project directory structure
tree -I "vendor"