What are namespaces?
Namespaces are a way of encapsulating items.
e.g. In any operating system directories serve to group related files, and act as a namespace for the files within them.
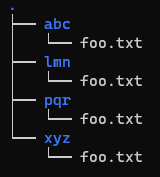
As a concrete example, the file foo.txt
can exist in all 4 directories with different contents in each.
Similarly to avoid ambiguity in PHP we have namespaces. Namespaces solve the confusion of having same class name in program.
Let’s understand this with example.
AdminAccount.php
<?php
class AdminAccount {
public function __construct() {
echo "Admin Account Controller..." . PHP_EOL;
}
}
UserAccount.php
<?php
class UserAccount {
public function __construct() {
echo "User Account Controller..." . PHP_EOL;
}
}
index.php
<?php
require("UserAccount.php");
require("AdminAccount.php");
new UserAccount();
new AdminAccount();
Now, what if we have the same class name in both files.
This scenario always comes when you use third-party libraries or any framework.
AdminAccount.php
<?php
class Account {
public function __construct() {
echo "Admin Account Controller..." . PHP_EOL;
}
}
UserAccount.php
<?php
class Account {
public function __construct() {
echo "User Account Controller..." . PHP_EOL;
}
}
index.php
<?php
require("UserAccount.php");
require("AdminAccount.php");
new Account();
new Account();
This will create confusion about which instance to be created.
Now let’s resolve this problem using namespaces.
AdminAccount.php
<?php
namespace Admin;
class Account {
public function __construct() {
echo "Admin Account Controller..." . PHP_EOL;
}
}
UserAccount.php
<?php
namespace User;
class Account {
public function __construct() {
echo "User Account Controller..." . PHP_EOL;
}
}
index.php
<?php
require("UserAccount.php");
require("AdminAccount.php");
new User\Account();
new Admin\Account();